Predicting New Images#
Requirements#
Here we gather the required libraries, classes and function for this notebook.
from PIL import Image
import os
import sys
import pickle
PyImageML
is a Python package that has been developed under this project, which has several utils for plotting images and extracting features from them, features that later could be used along with Machine Learning algorithms to solve typical ML tasks.
sys.path.insert(0, r"C:\Users\fscielzo\Documents\Packages\PyImageML_Package_Private")
from PyImageML.preprocessing import ImageFeaturesExtraction
PyMachineLearning
is another custom Python package that contains efficient utils to be used in real Machine Learning workflows.
sys.path.insert(0, r'C:\Users\fscielzo\Documents\Packages\PyMachineLearning_Package_Private')
from PyMachineLearning.preprocessing import scaler, pca
In this section new images will be classified using the best found pipeline as it was saved before.
# Load the model from file
with open(r'C:\Users\fscielzo\Documents\DataScience-GitHub\Image Analysis\Image-Classification\Fire-Detection\Results\best_pipeline.pkl', 'rb') as file:
loaded_pipeline = pickle.load(file)
# Building a list with the new images paths
folder_new_images = r'C:\Users\fscielzo\Documents\DataScience-GitHub\Image Analysis\Image-Classification\Fire-Detection\New-Data'
new_images_names = os.listdir(folder_new_images)
new_images_paths = [os.path.join(folder_new_images, file_name) for file_name in new_images_names]
# Get all files in the folder
X_new = new_images_paths
# Predicting the new images with the loaded pipeline
Y_new_hat = loaded_pipeline.predict(X_new)
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 260ms/step
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 132ms/step
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 126ms/step
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 143ms/step
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 174ms/step
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 130ms/step
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 134ms/step
1/1 ━━━━━━━━━━━━━━━━━━━━ 0s 147ms/step
Plotting the images along with the model predictions.
for i, (img, img_name) in enumerate(zip(X_new, new_images_names)):
print(f'--------------------------\nPredicting {img_name}\n')
if Y_new_hat[i] == 1:
print('Result: Fire detected\n--------------------------')
else:
print('Result: Fire not detected\n--------------------------')
display(Image.open(img).resize((500, 350)))
--------------------------
Predicting img1.png
Result: Fire detected
--------------------------
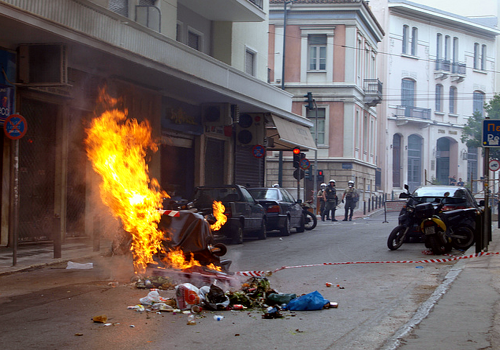
--------------------------
Predicting img2.jpg
Result: Fire detected
--------------------------
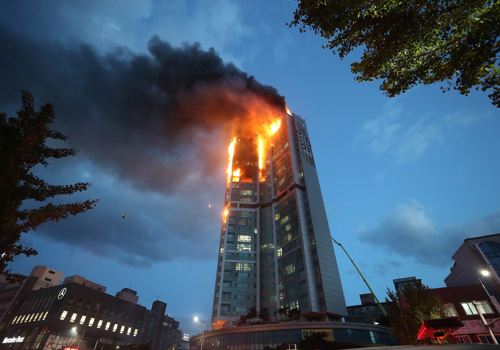
--------------------------
Predicting img3.jpg
Result: Fire detected
--------------------------
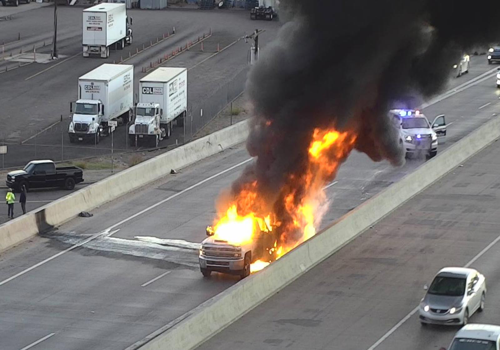
--------------------------
Predicting img4.jpg
Result: Fire not detected
--------------------------
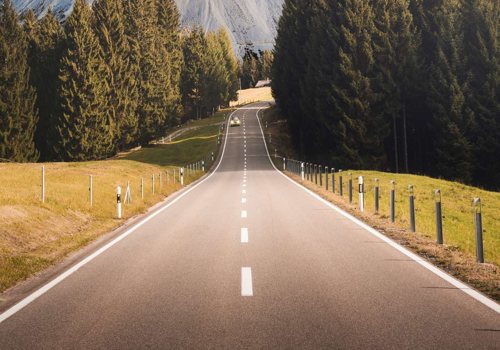
--------------------------
Predicting img5.jpg
Result: Fire not detected
--------------------------
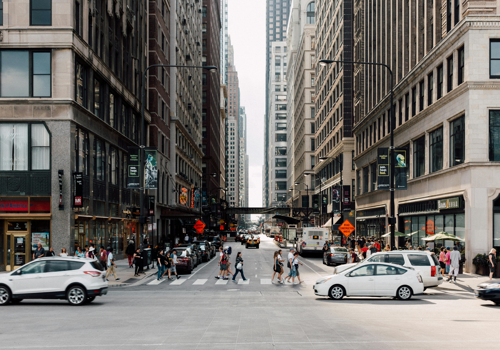
--------------------------
Predicting img6.jpg
Result: Fire not detected
--------------------------
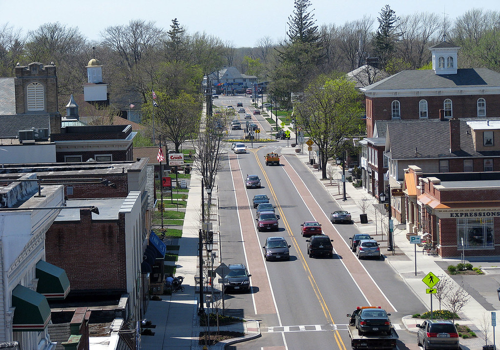
--------------------------
Predicting img7.jpg
Result: Fire not detected
--------------------------
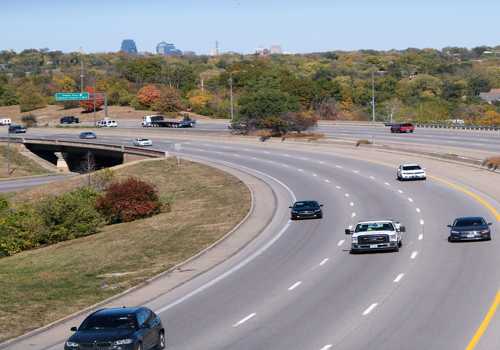
--------------------------
Predicting img8.jpg
Result: Fire detected
--------------------------
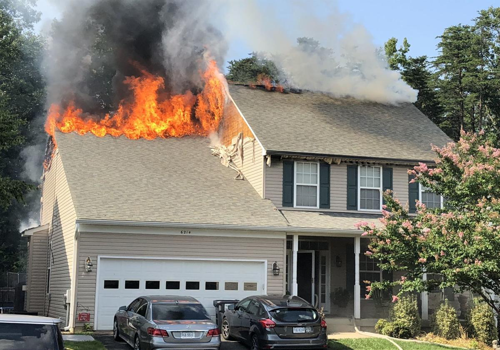
All the new images have been classified correctly.